Mastering Worksheets with VBA
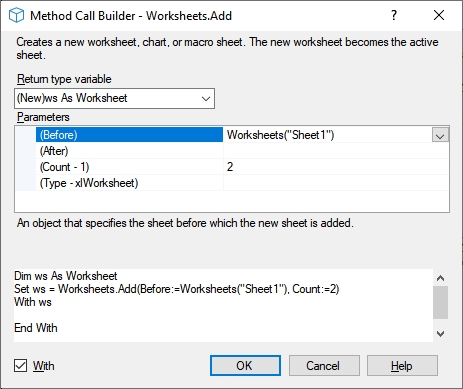
Unlocking the Power of Worksheets with VBA
Worksheets are a fundamental component of Microsoft Excel, and when combined with Visual Basic for Applications (VBA), they become an even more powerful tool for data analysis and manipulation. In this article, we will explore the world of worksheets with VBA, covering the basics, advanced techniques, and best practices to help you unlock the full potential of your worksheets.
Getting Started with Worksheets and VBA
Before diving into the world of worksheets with VBA, it’s essential to understand the basics of both components. A worksheet is a single page within an Excel workbook, where you can enter and manipulate data. VBA, on the other hand, is a programming language that allows you to automate tasks, interact with Excel objects, and create custom tools.
To start working with worksheets and VBA, you’ll need to:
- Open the Visual Basic Editor (VBE) by pressing Alt + F11 or navigating to Developer > Visual Basic
- Create a new module by clicking Insert > Module
- Start writing your VBA code in the module window
Basic Worksheet Operations with VBA
VBA provides a wide range of methods and properties for interacting with worksheets. Here are some basic operations you can perform:
- Activate a worksheet:
Worksheets("Sheet1").Activate
- Select a range:
Range("A1:B2").Select
- Enter a value:
Range("A1").Value = "Hello World"
- Format a cell:
Range("A1").Font.Bold = True
These basic operations can be combined to perform more complex tasks, such as data manipulation and analysis.
Advanced Worksheet Techniques with VBA
Once you’ve mastered the basics, you can move on to more advanced techniques, such as:
- Looping through ranges:
For Each cell In Range("A1:B2")
- Using worksheet events:
Worksheet_Change(ByVal Target As Range)
- Creating custom worksheet functions:
Function MyFunction() As Variant
These advanced techniques allow you to automate complex tasks, interact with users, and extend the functionality of Excel.
Best Practices for Working with Worksheets and VBA
To ensure your worksheets and VBA code are efficient, readable, and maintainable, follow these best practices:
- Use meaningful variable names:
Dim myRange As Range
- Keep code organized:
Module1
for worksheet-specific code,Module2
for general functions - Test and debug code: Use the Immediate window,
Debug.Print
, and breakpoints to identify issues - Use worksheet-specific objects:
Worksheets("Sheet1")
instead ofActiveSheet
By following these best practices, you’ll be able to create robust, efficient, and maintainable worksheets with VBA.
Common Worksheet Errors and How to Avoid Them
When working with worksheets and VBA, it’s essential to be aware of common errors and how to avoid them:
- Error 1004: Method ‘Range’ of object ‘_Worksheet’ failed: Ensure the worksheet is active and the range is valid
- Error 91: Object variable or With block variable not set: Declare and initialize variables before using them
- Error 13: Type mismatch: Ensure variables and data types match
By understanding these common errors, you’ll be able to avoid frustration and ensure your worksheets and VBA code run smoothly.
Real-World Applications of Worksheets with VBA
Worksheets with VBA have numerous real-world applications, including:
- Data analysis and visualization: Automate data manipulation, create custom charts, and perform statistical analysis
- Business process automation: Streamline tasks, such as data entry, report generation, and email automation
- Scientific computing: Perform complex calculations, simulate models, and visualize results
By applying the techniques and best practices outlined in this article, you’ll be able to unlock the full potential of your worksheets with VBA and tackle a wide range of real-world challenges.
💡 Note: Always test and debug your VBA code to ensure it runs smoothly and efficiently.
Conclusion
In conclusion, worksheets with VBA are a powerful combination for data analysis, manipulation, and automation. By mastering the basics, advanced techniques, and best practices outlined in this article, you’ll be able to unlock the full potential of your worksheets and tackle a wide range of real-world challenges.
What is the difference between a worksheet and a workbook?
+A worksheet is a single page within an Excel workbook, where you can enter and manipulate data. A workbook, on the other hand, is a collection of worksheets.
How do I create a new module in the Visual Basic Editor?
+To create a new module, click Insert > Module in the Visual Basic Editor.
What is the purpose of the Immediate window in the Visual Basic Editor?
+The Immediate window allows you to test and debug code, execute VBA statements, and view the results.