Mastering VBA: Working with the Active Worksheet
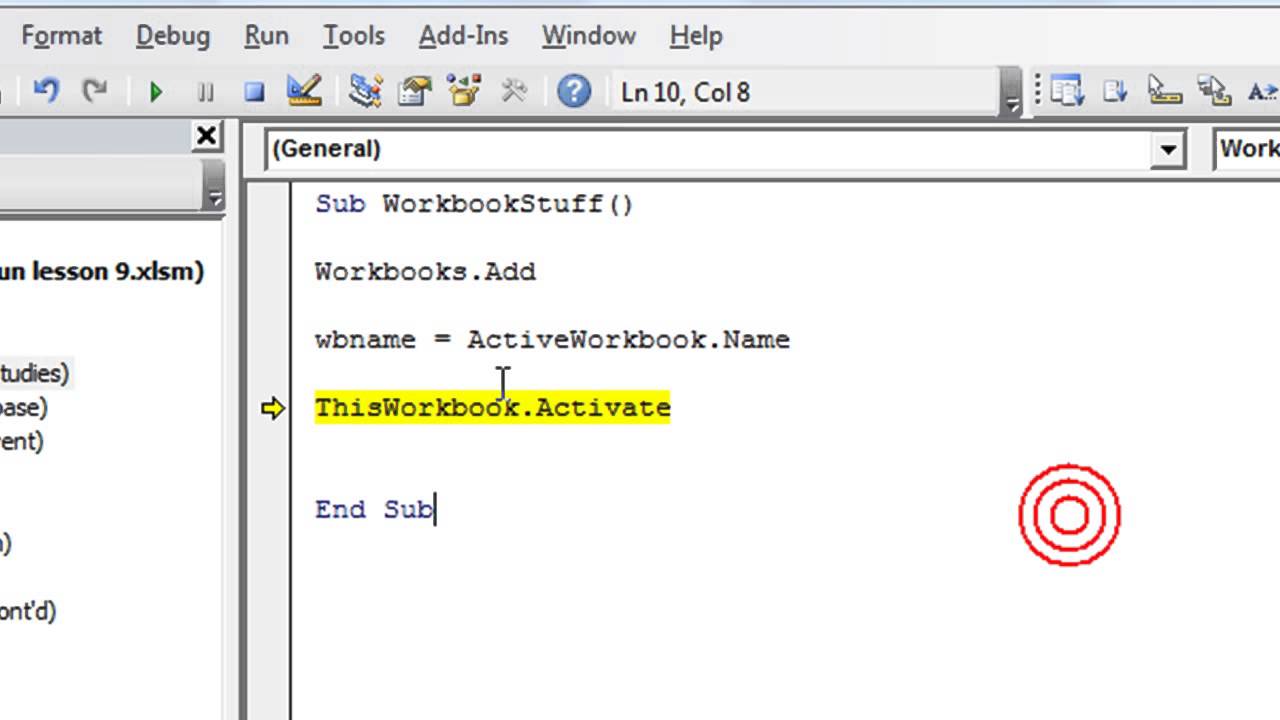
Working with the Active Worksheet in VBA
When working with Microsoft Excel, the active worksheet is the sheet that is currently being displayed and interacted with. In VBA, the active worksheet can be manipulated using various methods and properties. In this article, we will explore how to work with the active worksheet in VBA, including selecting cells, ranges, and manipulating worksheet properties.
Selecting Cells and Ranges in the Active Worksheet
To select cells and ranges in the active worksheet, you can use the Range
object. The Range
object represents a cell or a range of cells in the worksheet. You can use the Range
object to select a specific cell or range of cells using the Select
method.
Sub SelectCells()
' Select cell A1
Range("A1").Select
' Select range A1:B2
Range("A1:B2").Select
End Sub
You can also use the Cells
property to select a cell or range of cells. The Cells
property returns a Range
object that represents a cell or range of cells.
Sub SelectCells()
' Select cell A1
Cells(1, 1).Select
' Select range A1:B2
Range(Cells(1, 1), Cells(2, 2)).Select
End Sub
Manipulating Worksheet Properties
You can also manipulate worksheet properties using VBA. For example, you can change the worksheet name, hide or unhide the worksheet, and change the worksheet tab color.
Sub ManipulateWorksheetProperties()
' Change the worksheet name
ActiveSheet.Name = "MyWorksheet"
' Hide the worksheet
ActiveSheet.Visible = False
' Unhide the worksheet
ActiveSheet.Visible = True
' Change the worksheet tab color
ActiveSheet.Tab.ColorIndex = 3
End Sub
Working with Worksheet Events
Worksheet events are actions that occur when a user interacts with a worksheet, such as selecting a cell or changing a value. You can use VBA to capture these events and perform actions in response.
Private Sub Worksheet_SelectionChange(ByVal Target As Range)
' Code to run when the selection changes
MsgBox "Selection changed to " & Target.Address
End Sub
Private Sub Worksheet_Change(ByVal Target As Range)
' Code to run when the value changes
MsgBox "Value changed in " & Target.Address
End Sub
Best Practices for Working with the Active Worksheet
When working with the active worksheet in VBA, there are several best practices to keep in mind:
- Always use the
ActiveSheet
object to refer to the active worksheet, rather than relying on the worksheet name. - Use the
Range
object to select cells and ranges, rather than using theSelect
method. - Avoid using the
Select
method whenever possible, as it can slow down your code and cause errors. - Use worksheet events to capture user interactions and perform actions in response.
🔍 Note: Always use the `ActiveSheet` object to refer to the active worksheet, rather than relying on the worksheet name.
Common Errors When Working with the Active Worksheet
Here are some common errors to watch out for when working with the active worksheet:
- Error 1004: Method ‘Range’ of object ‘_Worksheet’ failed. This error occurs when you try to select a range that is not valid.
- Error 424: Object required. This error occurs when you try to use the
ActiveSheet
object without first selecting a worksheet.
🚨 Note: Always check the worksheet name and range before trying to select it.
Conclusion
In this article, we explored how to work with the active worksheet in VBA, including selecting cells and ranges, manipulating worksheet properties, and working with worksheet events. By following best practices and avoiding common errors, you can write efficient and effective VBA code that interacts with the active worksheet.
What is the difference between the Range
object and the Cells
property?
+
The Range
object represents a cell or range of cells in the worksheet, while the Cells
property returns a Range
object that represents a cell or range of cells. The Cells
property is used to select a cell or range of cells using row and column numbers.
How do I capture worksheet events in VBA?
+
You can capture worksheet events in VBA by using worksheet event procedures, such as Worksheet_SelectionChange
and Worksheet_Change
. These procedures allow you to write code that runs when a user interacts with the worksheet.
What is the best practice for referring to the active worksheet in VBA?
+
The best practice is to use the ActiveSheet
object to refer to the active worksheet, rather than relying on the worksheet name.
Related Terms:
- Workbook worksheet vba
- Activate VBA Excel
- Excel VBA get sheet name
- Worksheet function VBA
- Workbook worksheet activate vba
- Workbooks activate VBA