5 Ways to Match Lowercase and Uppercase Letters

Understanding Letter Case and Its Importance
In the world of text processing and data analysis, the case of letters can significantly affect the outcome of various operations. Whether you’re working with programming languages, data entry, or text manipulation tools, understanding how to handle uppercase and lowercase letters is crucial. This post will explore five ways to match lowercase and uppercase letters, ensuring that you can work efficiently with text data.
Method 1: Using Case-Insensitive Matching in Programming Languages
Most programming languages provide ways to perform case-insensitive string matching. This method involves ignoring the case of letters during comparisons, allowing you to match strings regardless of their case.
# Python example
string1 = "Hello"
string2 = "hello"
if string1.casefold() == string2.casefold():
print("Strings match")
In this example, Python’s casefold()
method is used to convert both strings to a case-folded format, making the comparison case-insensitive.
Method 2: Utilizing Case Conversion Functions in Excel
Microsoft Excel offers various functions to convert text to uppercase or lowercase. You can use these functions to standardize text data and make it easier to match.
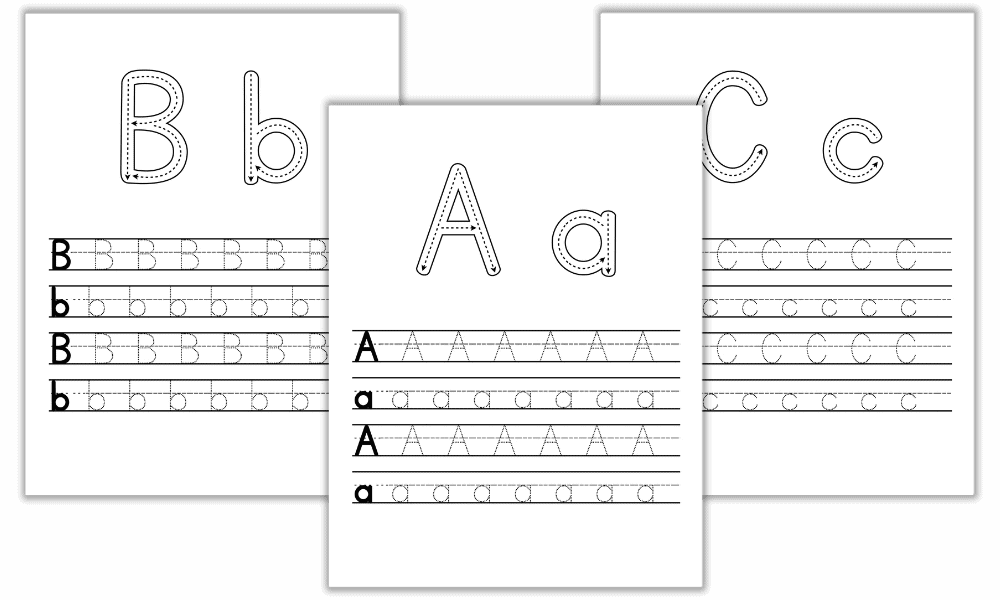
Formula | Description |
---|---|
UPPER(A1) |
Converts the text in cell A1 to uppercase |
LOWER(A1) |
Converts the text in cell A1 to lowercase |
PROPER(A1) |
Converts the text in cell A1 to proper case (first letter capitalized) |
By applying these functions to your text data, you can easily match strings regardless of their original case.
Method 3: Leveraging Regular Expressions (Regex) for Pattern Matching
Regular expressions provide a powerful way to match patterns in text data, including case-insensitive matching. By using the (?i)
flag or the i
modifier, you can make your regex patterns case-insensitive.
// JavaScript example
const regex = /hello/i;
const string = "HELLO";
if (regex.test(string)) {
console.log("String matches");
}
In this example, the i
flag makes the regex pattern case-insensitive, allowing it to match the string “HELLO” despite the difference in case.
Method 4: Using SQL Queries with Case-Insensitive Matching
When working with databases, you can use SQL queries to match strings regardless of their case. Most database management systems provide functions or modifiers to perform case-insensitive matching.
-- SQL example
SELECT * FROM table_name
WHERE LOWER(column_name) = 'hello';
In this example, the LOWER()
function converts the column values to lowercase, allowing the query to match strings regardless of their original case.
Method 5: Employing Text Manipulation Tools with Case-Insensitive Options
Text manipulation tools like sed
and awk
often provide options for case-insensitive matching. By using these options, you can easily match strings regardless of their case.
# sed example
sed -i 's/Hello/hello/I' file.txt
In this example, the I
flag makes the substitution case-insensitive, allowing sed
to replace all occurrences of “Hello” (regardless of case) with “hello”.
👍 Note: The availability of case-insensitive options may vary depending on the specific tool or programming language being used.
When working with text data, it’s essential to consider the case of letters to ensure accurate matching and processing. By utilizing the methods outlined in this post, you can efficiently match lowercase and uppercase letters in various contexts.
Maintaining consistent case handling is crucial for efficient text processing, data analysis, and programming. Whether you’re working with programming languages, data entry, or text manipulation tools, understanding how to handle uppercase and lowercase letters is vital for achieving accurate results.