5 Ways to Identify Functions Easily
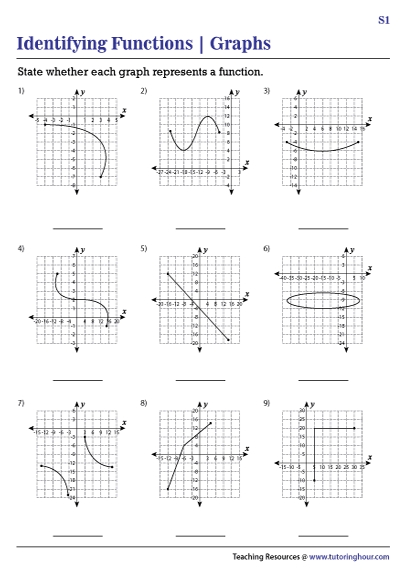
Understanding Functions: The Building Blocks of Programming
When it comes to programming, functions are the fundamental units of code that perform specific tasks. They are reusable blocks of code that take in arguments, process them, and return a value. Identifying functions is crucial for any programmer, as it helps in understanding the code’s logic and functionality. In this article, we will explore five ways to identify functions easily.
1. Look for Function Keywords
One of the easiest ways to identify functions is to look for specific keywords that are used to define them. In most programming languages, functions are defined using keywords such as function
, def
, sub
, or proc
. For example, in JavaScript, functions are defined using the function
keyword, while in Python, they are defined using the def
keyword.
Here’s an example of a function definition in JavaScript:
function add(x, y) {
return x + y;
}
And here’s an example of a function definition in Python:
def add(x, y):
return x + y
2. Check for Argument Lists
Functions typically take in arguments, which are values passed to the function when it is called. These arguments are usually enclosed in parentheses and separated by commas. By looking for argument lists, you can identify potential functions.
Here’s an example of a function with an argument list in Java:
public int add(int x, int y) {
return x + y;
}
3. Look for Return Statements
Functions often return values, which are specified using the return
statement. By looking for return statements, you can identify functions that produce output.
Here’s an example of a function with a return statement in C++:
int add(int x, int y) {
return x + y;
}
4. Check for Function Calls
Functions are often called from other parts of the code. By looking for function calls, you can identify potential functions. Function calls typically involve the function name followed by an argument list in parentheses.
Here’s an example of a function call in PHP:
$result = add(2, 3);
5. Analyze the Code Structure
Finally, analyzing the code structure can help you identify functions. Functions are typically defined in a specific format, with a clear beginning and end. By looking at the code structure, you can identify potential functions.
Here’s an example of a code structure that suggests a function in Ruby:
def add(x, y)
# code here
end
💡 Note: The code structure may vary depending on the programming language and style.
Conclusion
Identifying functions is a crucial skill for any programmer. By looking for function keywords, argument lists, return statements, function calls, and analyzing the code structure, you can easily identify functions in your code. Remember, functions are the building blocks of programming, and understanding them is essential for writing efficient and effective code.
What is a function in programming?
+A function is a block of code that performs a specific task and can be reused throughout the program.
How do I define a function in JavaScript?
+In JavaScript, you define a function using the function
keyword followed by the function name and argument list.
What is the purpose of a return statement in a function?
+The return statement specifies the value that the function returns to the caller.