5 Ways to Master Function Composition
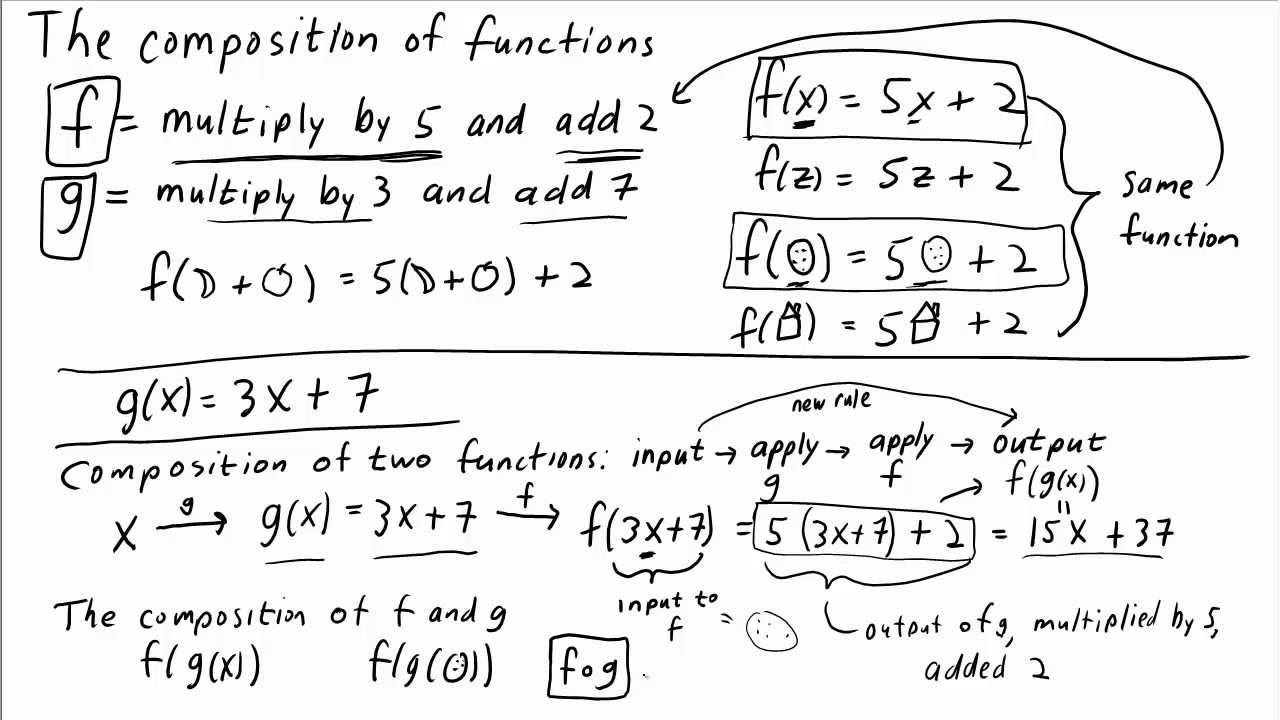
Understanding Function Composition
Function composition is a fundamental concept in programming that involves combining two or more functions to create a new function. This technique is widely used in functional programming languages, such as Haskell and Lisp, but itโs also applicable in other programming paradigms. Mastering function composition can help you write more concise, readable, and maintainable code.
What is Function Composition?
Function composition is the process of creating a new function by combining two or more existing functions. The new function, often called the composed function, takes the output of one function as the input for another function. This process can be repeated multiple times, allowing you to create complex functions from simpler ones.
Benefits of Function Composition
Function composition offers several benefits, including:
- Improved code readability: By breaking down complex functions into smaller, more manageable pieces, you can make your code easier to understand and maintain.
- Increased modularity: Function composition allows you to reuse code and create new functions by combining existing ones, making your code more modular and flexible.
- Reduced bugs: By minimizing the complexity of individual functions, you can reduce the likelihood of errors and make debugging easier.
5 Ways to Master Function Composition
To master function composition, follow these five techniques:
1. Understand the Basics of Function Composition
Before diving into advanced techniques, make sure you understand the basics of function composition. A composed function takes the output of one function as the input for another function. For example:
function add(x) {
return x + 1;
}
function multiply(x) {
return x * 2;
}
function compose(add, multiply) {
return function(x) {
return multiply(add(x));
}
}
const composed = compose(add, multiply);
console.log(composed(5)); // Output: 12
In this example, the compose
function takes two functions, add
and multiply
, and returns a new function that applies multiply
to the result of add
.
2. Use Higher-Order Functions
Higher-order functions are functions that take other functions as arguments or return functions as output. They are essential for function composition. For example:
function curry(fn) {
return function(...args) {
if (args.length < fn.length) {
return curry(fn.bind(null,...args));
}
return fn(...args);
}
}
function add(x, y) {
return x + y;
}
const curriedAdd = curry(add);
console.log(curriedAdd(2)(3)); // Output: 5
In this example, the curry
function takes a function fn
and returns a new function that can be called with fewer arguments. The curriedAdd
function is created by applying curry
to the add
function.
3. Utilize Function Libraries
There are several function libraries available that provide implementations of common functions, such as map
, filter
, and reduce
. These libraries can help you write more concise and readable code. For example:
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5];
const doubleNumbers = _.map(numbers, x => x * 2);
console.log(doubleNumbers); // Output: [2, 4, 6, 8, 10]
In this example, the lodash
library is used to create a new array with the numbers doubled.
4. Learn to Debug Composed Functions
Debugging composed functions can be challenging, but there are several techniques to help you. One approach is to use the console.log
function to output intermediate results. For example:
function compose(add, multiply) {
return function(x) {
const result = add(x);
console.log(`Result of add: ${result}`);
return multiply(result);
}
}
const composed = compose(add, multiply);
console.log(composed(5));
In this example, the console.log
function is used to output the intermediate result of the add
function.
5. Practice, Practice, Practice
The best way to master function composition is to practice writing composed functions. Start with simple examples and gradually move on to more complex ones. Try to combine different functions to create new ones, and experiment with different libraries and techniques.
๐ Note: Function composition is a fundamental concept in programming, and mastering it can help you write more concise, readable, and maintainable code.
By following these five techniques, you can improve your skills in function composition and become a more proficient programmer.
Summing up, function composition is a powerful technique that can help you write more efficient and maintainable code. By understanding the basics, using higher-order functions, utilizing function libraries, learning to debug composed functions, and practicing regularly, you can master function composition and take your programming skills to the next level.
What is function composition?
+Function composition is the process of creating a new function by combining two or more existing functions.
What are the benefits of function composition?
+The benefits of function composition include improved code readability, increased modularity, and reduced bugs.
How can I master function composition?
+You can master function composition by understanding the basics, using higher-order functions, utilizing function libraries, learning to debug composed functions, and practicing regularly.